Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |
Tags
- 공동작업자
- 구성파일
- aws
- VSCode
- linux Xshell
- Linux
- Github
- oracle vm virtualbox
- VSCode업로드
- Spring
- insert안됨
- html템플릿
- EaaS
- 코테관련공부
- react-dom
- SpringBoot개발환경
- 명령어 모음
- 편집모드
- GiyHub
- Function
- react
- 코딩애플
- javascript
- java.sql.SQLException: Incorrect string value: #RDS #AWS #mariadb #springboot
- 사용자 권한 부여
- Biling and Cost Manager
- Disk추가
- AWS #AWS장단점 #AWS차별화 # AWS서비스
- Push
- OracleVMVirtualBox
Archives
- Today
- Total
귀농 전까지 쓰는 개발 일지
[JavaScript] 자료형 본문
(1) 변수(Variable)
- let: 변수명 똑같이 중복 선언 '불가' / 중괄호 사용 시 범위가 한정적 (지역변수)
let title = '제목';
console.log(title); //Console창에 출력
title = '제목수정';
console.log(title);
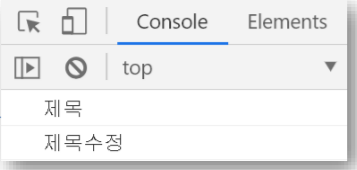
let globalScope = 'global name';
{
let name = 'javascript'; //let 변수
console.log(name);
name = 'hello';
console.log(name);
}
console.log(name);
console.log(globalScope);
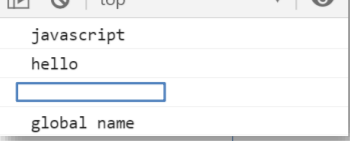
- var : 변수명 똑같이 중복 선언 '가능' / 중괄호 내 사용 시 범위 한정적X
let globalScope = 'global name';
{
var name = 'javascript'; //var 변수
console.log(name);
name = 'hello';
console.log(name);
}
console.log(name);
console.log(globalScope);
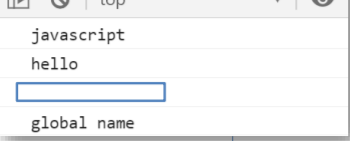
- Constants(상수) : 입력값 수정 불가
const title = '제목';
title = '제목수정'; //Uncaught TypeError
(2) Hoisting(호이스팅)
- 실제 선언 위치와 관계없이 코드의 위로 끌어올려 선언 / 값 할당은 실제 위치에서 동작
//var
value = 10;
console.log(value); // ==========> 정상동작!!! value: 10 출력
var value;
//let
value = 10;
console.log(value); //Uncaught ReferenceError
let value;
(3) Variable Type
- Primitive (기본): 더 이상 나눠지지 않는 값 -> number, string, boolean, null, undefined, symbol
- Reference (참조): Single item 들을 하나의 단위로 관리 -> { }, [ ], class
- Function (함수): First-calss Function / 함수를 다른 변수와 동일하게 다룸
(3)-1. Primitive(기본)
- number
<script>
const num = 17; //integer
const num2 = 17.1; //decimal number
console.log(`num value: ${num}, type: ${typeof num}`);
console.log(`num2 value: ${num2}, type: ${typeof num2}`);
const infinity = 1 / 0; //양의 무한대
const negativeInfinaty = -1 / 0; //음의 무한대
console.log(`infinity value: ${infinity}, type: ${typeof infinity}`);
console.log(`negativeInfinaty value: ${negativeInfinaty}, type: ${typeof negativeInfinaty}`);
const nan = 'not a number' / 2; //숫자가 아닌 값
console.log(`nan value: ${nan}, type: ${typeof nan}`);
//*** 숫자인지 아닌지 판별 ***
isNaN("isnan"); //true출력 -> 숫자X true, 숫자O false
isNaN(true); //false출력 -> boolean형도 숫자로 취급함 true:1, false:0
</script>
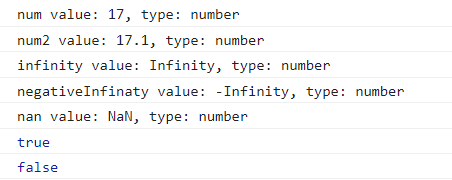
- string
<h1>String사용법</h1>
<body>
<script>
const a = 'string ex';
console.log(`a value: ${a}, type: ${typeof a}`);
//문자열 + 문자열
const addString = a + '문자 더하기'
console.log(`addString value:${addString}, type: ${typeof addString}`);
const addString2 = `${a} 문자 더하기2! `;
console.log(`addString2 value:${addString2}, type:${typeof addString2}`);
</script>
</body>

* string(indexing/함수): str.length / str.substring(0,4) / str.slice(-6, str.length) ...
- boolean
false : 0, null, undefind, NaN, ' '
true : false가 아닌 값
- null / undefined
null : 값을 할당하지 않았음을 명시
undefined : 선언만 하고 값에 대해 언급X
(3)-2. Reference(참조)
- Object
<h1>Reference</h1>
<body>
<script>
const obj1 = { name: 'hoing', age: 23};
console.log(`변경전: ${obj1.age}`);
obj1.age = 24;
console.log(`변경후: ${obj1.age}`);
const arr = [ 1,2,3];
arr[0]= 10;
for(let i=0; i<arr.length; i++) console.log(arr[i]); ;
</script>
</body>
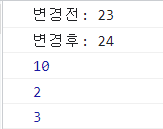
'공부 > JavaScript' 카테고리의 다른 글
<JavaScript> 2차원 배열 선언 / 정렬(Sort) (0) | 2023.01.29 |
---|---|
[JavaScript] 배열 (0) | 2022.01.17 |
[JavaScript] function(3) - IIFE / Closure (0) | 2022.01.17 |
[JavaScript] function(2) - 활용, Arrow Function (0) | 2022.01.17 |
[JavaScript] function(1)-형태/기본값/가변인자 (0) | 2022.01.17 |